Physical Modeling Project Update
So far for my project I have read, and read and read, and now after some implementation, I have come to an understanding of how the general framework for modal analysis works.
Basically, there is a set of eigenfunctions (in the continuum) or eigenvectors (discretize), along with a set of weights on each eigenfunction. This forms a basis (as the functions are orthogonal) which determines the shape of the object being modeled. As the simulation progresses the weights change (but not the eigenfunctions). The change of the weights with respect to time is the pressure (which is the sound).
For a circular membrane, the eigenfuncitons are defined by the bessel function and its zeros, but for shapes where this is not know this must be pre-computed.
So putting the pieces together, the general sound rendering loop for modal analysis works by updating the weights at every time step, next scaling the eigenfunctions by the weights, and finally summing up the scaled eigenfunctions to form the displacements. As stated earlier this change of weight is the sound.
So far I have started doing some coding in matlab and mathematica to quickly see what it is that is going on, and get a better idea of how this all works. The pictures were generated by first pre-computing the zeros for the bessel function in mathmatica. I did this for bessel funcitons order [0,4], and calculated their first 4 roots each. Next I visualized the eigenfunctions for a membrane for various bessel functions with different number of roots. Finally I created a set of weights and created a weighted sum of eigenfunctions. All of this can be found below in the Matlab report.
Contents
Define mesh grid for program usage
[x,y]=meshgrid(-1:0.05:1,-1:0.05:1);
Bessel order 0 with root 1
i=0; j=1;
surf(x,y,f_mn_2(i,j,x,y,0));
str = sprintf('Bessel order %i Root %i',i,j);
title(str);

Bessel order 1 with root 1
i=1; j=1;
surf(x,y,f_mn_2(i,j,x,y,0));
str = sprintf('Bessel order %i Root %i',i,j);
title(str);

Bessel order 4 with root 1
i=4; j=1;
surf(x,y,f_mn_2(i,j,x,y,0));
str = sprintf('Bessel order %i Root %i',i,j);
title(str);

Bessel order 1 with root 4
i=1; j=4;
surf(x,y,f_mn_2(i,j,x,y,0));
str = sprintf('Bessel order %i Root %i',i,j);
title(str);

Bessel order 3 with root 4
i=3; j=4;
surf(x,y,f_mn_2(i,j,x,y,0));
str = sprintf('Bessel order %i Root %i',i,j);
title(str);

Summation and scalling of multiple Eigenfunctions
calulate z for each eigen function
z01 = f_mn_2(0,1,x,y,0); z11 = f_mn_2(1,1,x,y,0); z41 = f_mn_2(4,1,x,y,0); z14 = f_mn_2(1,4,x,y,0); z34 = f_mn_2(3,4,x,y,0); % scaling factors a01 = .34; a11 = .22; a41 = .78; a14 = .18; a34 = .67; % scalez01 = f_mn_2(0,1,x,y,0); z01 = a01*z01; z11 = a11*z11; z41 = a41*z41; z14 = a14*z14; z34 = a34*z34; % sum all zi z = z01+z11+z41+z14+z34; surf(x,y,z); title('Weighted sum of eigenfunctions');
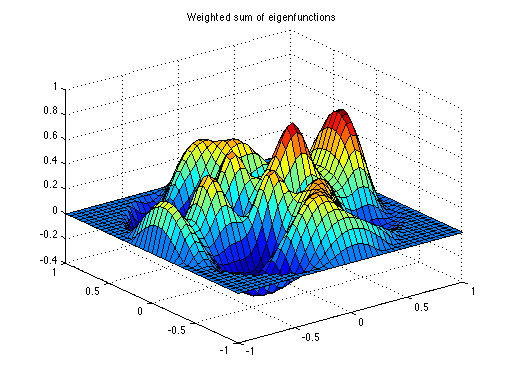